GET and SET field values using JavaScript in Power Pages
Introduction: In this article, you will learn how to GET and SET field values of different data types in power pages, power portals, and dynamic CRM portals using JavaScript.
To get and set field values of different data types using jQuery, you can utilize jQuery selectors and methods.
Getting Field Values
To retrieve the value of a field or element using jQuery, you can use the .val()
method. For example, if you have an input field with an id of “myInput”, you can get its value using jQuery as follows:
// Get the value of an input field
var inputValue = $("#myInput").val();
Here, $("#myInput")
selects the element with the specified id, and .val()
retrieves its current value.
Setting Field Values
To assign a value to a field or element using jQuery, you can also use the .val()
method. For example, if you want to set a value to an HTML element with an id of “myElement”, you can use jQuery like this:
// Set the value of an HTML element
$("#myInput").val("Hello, World!");
In this case, $("#myInput")
selects the element with the specified id, and .val("Hello, World!")
sets its value to the given string.
jQuery can handle different data types, including strings, numbers, booleans, arrays, and objects. You can manipulate these types using appropriate methods and operators.
Here’s an example of setting and getting field values for different data types using jQuery:
Text Field
GET
//Name is field ID
var accountName= $("#name").val();
SET
//Set Field Value
$("#name").val("TestuserAccountName");
Option Set
GET
//address1 addresstypecode is field ID
var addressType= $("#address1_addresstypecode").val();
SET

//Set Field Value
$("#address1_addresstypecode").val(2);
Radio Button
Two controls are rendered for the radio button. The syntax to read which radio button has been selected is listed below.
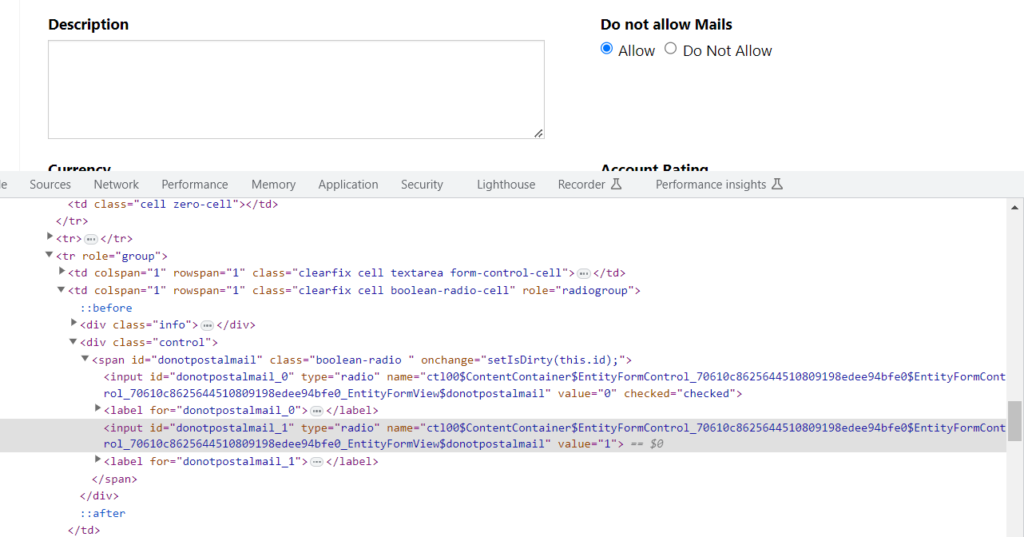
GET
var isAllowChecked = $("#donotpostalmail_0").is(":checked"); //Returns true/false
var isDoNotAllowChecked = $("#donotpostalmail_1").is(":checked"); //Returns true/false
SET
// To set Radio 1 to 'true'
$("#donotpostalmail_0").prop("checked", true);
// To set Radio 1 to 'false'
$("#donotpostalmail_0").prop("checked", false);
// To set Radio 2 to 'true'
$("#donotpostalmail_1").prop('checked', true);
// To set Radio 2 to 'false'
$("#donotpostalmail_1").prop("checked", false);
Look up
GET
var lookupGUID = $("#transactioncurrencyid").val(); // transactioncurrencyid is field id - Retruns GUID
var lookupValue = $("#transactioncurrencyid_name").val(); // Retruns Selcted Field Value
var entityName= $("#transactioncurrencyid_entityname").val(); // Returns Entity Name
SET
// transactioncurrencyid is filed ID
$("#transactioncurrencyid").val(lookupId); // lookupId -- GUID
$("#transactioncurrencyid_name").val(lookupName); // Record Value
$("#transactioncurrencyid_entityname").val(EntitySchemaName); // Entity Name
Date field in Portals
GET Date Field Value

var lastOnholdTime= $("#lastonholdtime").val(); // Result '2023-07-09T11:05:00.0000000Z'
// Convert to date
var formattedDate=moment(lastOnholdTime).format("DD-MM-YYYY"); //In Portals moment JS Library is included.
SET Date field Value
var dateValue = new Date(); // Today Date
// Get date field
var dateField = $("#lastonholdtime"); // lastonholdtime is field Id
var $displayField = dateField.nextAll(".datetimepicker").children("input");
var dateFormat = $displayField.attr("data-date-format");
dateField.val(moment.utc(dateValue).format("YYYY-MM-DDTHH:mm:ss.0000000Z"));
$displayField.val(moment(dateValue).format(dateFormat));
For more details on the date picker controls , please click on the below-mentioned article.
Checkbox
Get Checkbox Value

var isChecked = $("#donotsendmm").is(":checked");
if (isChecked == true) {
console.log("Checked!");
}
else{
console.log("UnChecked");
}
Remember to replace the field ids (“transactioncurrencyid”, “donotsendmm”, etc.) with the actual ids of your HTML elements.